Using dangerouslySetInnerHTML Safely in Next.js
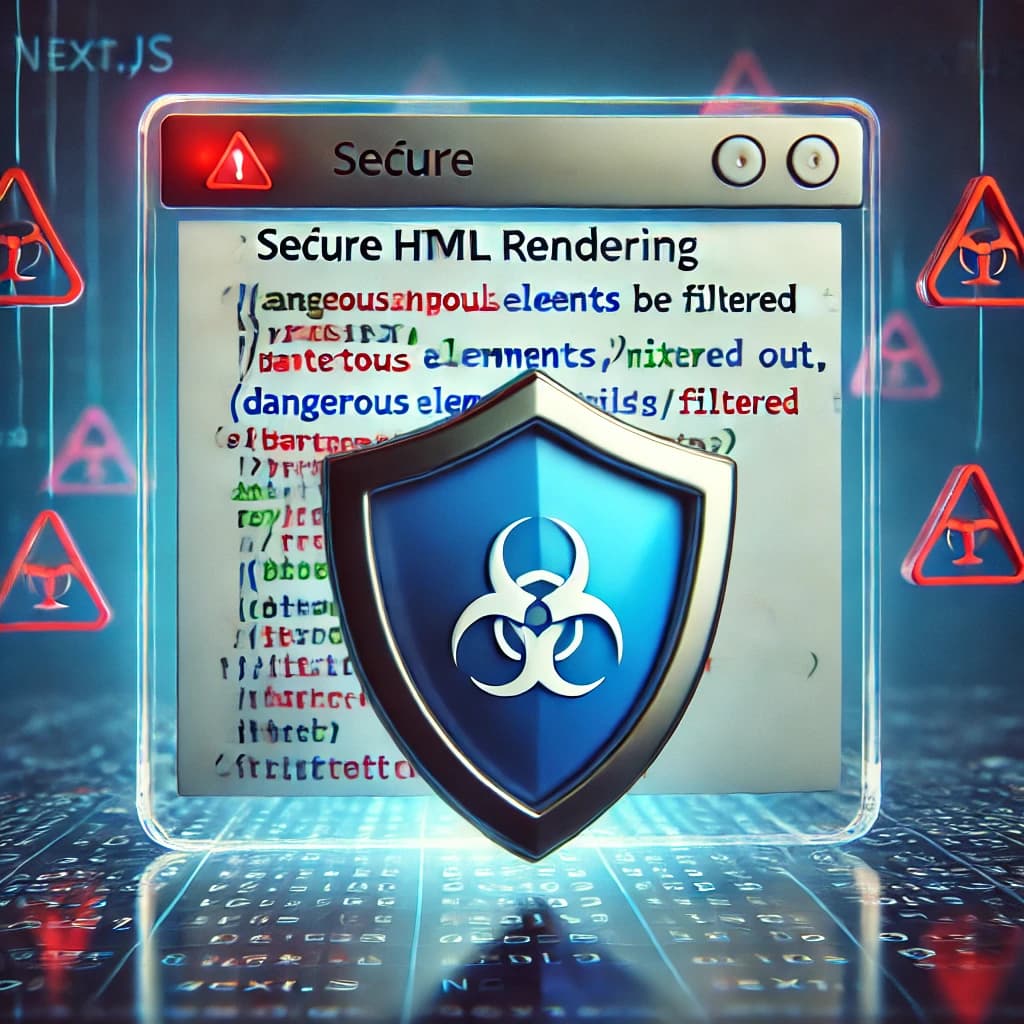
Using dangerouslySetInnerHTML Safely in NextJs with isomorphic-dompurify
If you’ve been working with Next.js, you’ve probably run into a situation where you need to render raw HTML inside a React component. Maybe you’re dealing with user-generated content or embedding third-party widgets. That’s where dangerouslySetInnerHTML
comes in—but as the name suggests, it’s not something you should use without caution.
Why is dangerouslySetInnerHTML
Dangerous?
React escapes content by default to prevent cross-site scripting (XSS) attacks. But when you use dangerouslySetInnerHTML
, you’re telling React to insert raw HTML into the DOM, which can be a security risk if the content isn’t sanitized properly.
For example, if you render user input directly using dangerouslySetInnerHTML
, a malicious user could inject harmful scripts:
const UnsafeComponent = ({ content }) => (
<div dangerouslySetInnerHTML={{ __html: content }} />
);
If content
contains something like:
<script>alert('Hacked!');</script>
Boom! That script will execute, potentially compromising your site and users.
How to Use dangerouslySetInnerHTML
Safely with isomorphic-dompurify
The good news is that you can still use dangerouslySetInnerHTML
safely by sanitizing the HTML first. One of the best ways to do this in a Next.js project is by using isomorphic-dompurify
.
Step 1: Install isomorphic-dompurify
Run the following command to add it to your project:
npm install isomorphic-dompurify
Or if you’re using yarn:
yarn add isomorphic-dompurify
Step 2: Sanitize Your HTML Before Rendering
Import the library and use it to clean up the HTML before passing it into dangerouslySetInnerHTML
:
import DOMPurify from 'isomorphic-dompurify';
const SafeComponent = ({ content }) => {
const cleanHTML = DOMPurify.sanitize(content);
return <div dangerouslySetInnerHTML={{ __html: cleanHTML }} />;
};
Now, even if someone tries to sneak in a <script>
tag, isomorphic-dompurify
will strip it out, making sure your application stays secure.
Why isomorphic-dompurify
?
You might be wondering, why use isomorphic-dompurify
instead of just dompurify
? The reason is simple: isomorphic-dompurify
works in both browser and server-side environments, making it perfect for Next.js applications where code can be executed on the server during rendering.
Extra Security Tips
While sanitizing HTML is a great first step, here are a few additional best practices to keep your Next.js app secure:
- Avoid Inline Event Handlers – Even with sanitization, avoid using inline event handlers like
onclick="alert('test')"
in your HTML content. - Set a Content Security Policy (CSP) – Use a strict CSP to further mitigate XSS risks.
- Validate User Input – Don’t just sanitize on the frontend; validate and sanitize input at the backend as well.
- Keep Dependencies Updated – Regularly update packages like
isomorphic-dompurify
to ensure you have the latest security patches.
Using dangerouslySetInnerHTML
in Next.js can be risky, but with the right precautions—like sanitizing content with isomorphic-dompurify
—you can use it safely. This approach lets you take advantage of raw HTML rendering while keeping your app secure from XSS attacks.
If you’re interested in learning more about Next.js security best practices, check out the official Next.js Security documentation. Stay safe and happy coding!